Multi inputs DIC¶
This notebook shows how to use multi correlation of the same increment using different image.
Experimental protocol¶
The idea behind this is to access a better measurement and an error estimation for displacement field and strain field.
To do so during the experiement, 4 differents pictures are taken at each time step. Therefore for the correlation between \(t_1\) and \(t_2\) we can performed 16 correlations of different images inputs.
Then we will do some statistic to better eveluat the strain field and the associated error.
Load the data¶
import xarray_dic.loadDIC as xldd
import xarray_dic.xarray_dic as xd
import xarray_symTensor2d.xarray_symTensor2d as xsT
import os
import natsort
import numpy as np
import xarray as xr
import matplotlib.pyplot as plt
%matplotlib inline
adr='/data/FlorianFiguiere/GI06/DIC/DIC/Loading/Speakle_01/nm_analysis/gdr/'
res=0.015
unit_spa='millimeter'
time_step=0.005
unit_time='macro strain'
ds=xldd.multi_load(xldd.load7D,adr,res,time_step,unit_time=unit_time,unit_res=unit_spa)
ds
<xarray.Dataset> Dimensions: (d: 2, ncor: 16, sT: 6, time: 3, x: 168, y: 398) Coordinates: * x (x) float64 0.0 0.27 0.54 0.81 ... 44.28 44.55 44.82 45.09 * y (y) float64 0.0 0.27 0.54 0.81 ... 106.4 106.7 106.9 107.2 * time (time) float64 0.005 0.01 0.015 Dimensions without coordinates: d, ncor, sT Data variables: displacement (ncor, time, y, x, d) float64 -4.229 0.1801 ... 0.06294 0.5392 strain (ncor, time, y, x, sT) float64 0.002688 -0.1184 ... nan nan Attributes: unit_time: macro strain step_size: 0.015 unit_position: millimeter window_size: 18 path_dat: /data/FlorianFiguiere/GI06/DIC/DIC/Loading/Speakle_01/nm_... DIC_software: 7D
xarray.Dataset
- d: 2
- ncor: 16
- sT: 6
- time: 3
- x: 168
- y: 398
- x(x)float640.0 0.27 0.54 ... 44.55 44.82 45.09
array([ 0. , 0.27, 0.54, 0.81, 1.08, 1.35, 1.62, 1.89, 2.16, 2.43, 2.7 , 2.97, 3.24, 3.51, 3.78, 4.05, 4.32, 4.59, 4.86, 5.13, 5.4 , 5.67, 5.94, 6.21, 6.48, 6.75, 7.02, 7.29, 7.56, 7.83, 8.1 , 8.37, 8.64, 8.91, 9.18, 9.45, 9.72, 9.99, 10.26, 10.53, 10.8 , 11.07, 11.34, 11.61, 11.88, 12.15, 12.42, 12.69, 12.96, 13.23, 13.5 , 13.77, 14.04, 14.31, 14.58, 14.85, 15.12, 15.39, 15.66, 15.93, 16.2 , 16.47, 16.74, 17.01, 17.28, 17.55, 17.82, 18.09, 18.36, 18.63, 18.9 , 19.17, 19.44, 19.71, 19.98, 20.25, 20.52, 20.79, 21.06, 21.33, 21.6 , 21.87, 22.14, 22.41, 22.68, 22.95, 23.22, 23.49, 23.76, 24.03, 24.3 , 24.57, 24.84, 25.11, 25.38, 25.65, 25.92, 26.19, 26.46, 26.73, 27. , 27.27, 27.54, 27.81, 28.08, 28.35, 28.62, 28.89, 29.16, 29.43, 29.7 , 29.97, 30.24, 30.51, 30.78, 31.05, 31.32, 31.59, 31.86, 32.13, 32.4 , 32.67, 32.94, 33.21, 33.48, 33.75, 34.02, 34.29, 34.56, 34.83, 35.1 , 35.37, 35.64, 35.91, 36.18, 36.45, 36.72, 36.99, 37.26, 37.53, 37.8 , 38.07, 38.34, 38.61, 38.88, 39.15, 39.42, 39.69, 39.96, 40.23, 40.5 , 40.77, 41.04, 41.31, 41.58, 41.85, 42.12, 42.39, 42.66, 42.93, 43.2 , 43.47, 43.74, 44.01, 44.28, 44.55, 44.82, 45.09])
- y(y)float640.0 0.27 0.54 ... 106.7 106.9 107.2
array([ 0. , 0.27, 0.54, ..., 106.65, 106.92, 107.19])
- time(time)float640.005 0.01 0.015
array([0.005, 0.01 , 0.015])
- displacement(ncor, time, y, x, d)float64-4.229 0.1801 ... 0.06294 0.5392
array([[[[[-4.22888672e+00, 1.80051270e-01], [-4.22924973e+00, 1.79824219e-01], [-4.22970520e+00, 1.80490723e-01], ..., [-4.10983521e+00, 8.88574219e-02], [-4.10808289e+00, 8.76855469e-02], [-4.10934448e+00, 8.86083984e-02]], [[-4.22971848e+00, 2.11080322e-01], [-4.22959900e+00, 1.80421143e-01], [-4.22994186e+00, 1.78691411e-01], ..., [-4.10912384e+00, 8.97839355e-02], [-4.10747681e+00, 8.73999023e-02], [-4.10835205e+00, 8.80371094e-02]], [[-4.22855988e+00, 2.09289551e-01], [-4.22964111e+00, 1.89909668e-01], [-4.22973770e+00, 1.80307617e-01], ..., ... ..., [ 6.50985718e-02, 5.44898071e-01], [ 6.09173584e-02, 5.42131348e-01], [ 6.23931885e-02, 5.44433441e-01]], [[-2.98919678e-04, 5.43068390e-01], [ 2.50579834e-03, 5.45564346e-01], [ 6.05484009e-03, 5.46112289e-01], ..., [ 6.12286377e-02, 5.44089432e-01], [ 5.98333740e-02, 5.41605606e-01], [ 6.05218506e-02, 5.41739960e-01]], [[ 1.91345215e-04, 5.41533508e-01], [ 1.59484863e-03, 5.42924652e-01], [ 5.08575439e-03, 5.46004028e-01], ..., [ 6.21350098e-02, 5.42355652e-01], [ 6.04394531e-02, 5.41452942e-01], [ 6.29370117e-02, 5.39189758e-01]]]]])
- strain(ncor, time, y, x, sT)float640.002688 -0.1184 nan ... nan nan
array([[[[[ 2.68750754e-03, -1.18420780e-01, nan, -5.31571656e-02, nan, nan], [-1.91243808e-03, -3.10848560e-03, nan, -4.28239611e-04, nan, nan], [ 1.72417040e-03, 2.55351001e-03, nan, 7.09954009e-04, nan, nan], ..., [ 1.09959545e-03, -2.89316941e-03, nan, -3.76027264e-03, nan, nan], [ 8.64602393e-04, 1.14416284e-03, nan, -1.77038729e-03, nan, nan], [-4.31012968e-03, 2.23266822e-03, nan, 2.15010077e-04, nan, nan]], [[ 5.49710542e-03, -6.16272204e-02, nan, -5.23896329e-02, nan, nan], [ 2.00547650e-03, -1.71358441e-03, nan, -1.25631643e-02, nan, nan], [ 8.08011566e-04, -3.36635415e-03, nan, -1.64249900e-03, nan, nan], ... [-9.56306234e-04, 4.87374328e-03, nan, -5.15534310e-04, nan, nan], [-1.34613947e-03, 2.54653487e-03, nan, -2.36008130e-03, nan, nan], [ 3.49834515e-03, 9.90649872e-03, nan, 2.69389013e-04, nan, nan]], [[ 5.16817998e-03, 5.71001088e-03, nan, 2.14699190e-03, nan, nan], [ 1.14431428e-02, 9.61059891e-03, nan, 5.37718367e-03, nan, nan], [ 4.27755015e-03, -2.62090587e-04, nan, -1.07169710e-03, nan, nan], ..., [-3.61228967e-03, 6.49860688e-03, nan, -2.30854657e-03, nan, nan], [ 2.59083533e-03, 2.42613209e-03, nan, -6.29091822e-03, nan, nan], [ 1.80557847e-03, 9.82961617e-03, nan, -2.26120651e-03, nan, nan]]]]])
- unit_time :
- macro strain
- step_size :
- 0.015
- unit_position :
- millimeter
- window_size :
- 18
- path_dat :
- /data/FlorianFiguiere/GI06/DIC/DIC/Loading/Speakle_01/nm_analysis/gdr/
- DIC_software :
- 7D
Compute the average and standard deviation¶
ads=ds.dic.dic_average()
/home/chauvet/miniconda3/envs/basepy39/lib/python3.9/site-packages/numpy/lib/nanfunctions.py:1664: RuntimeWarning: Degrees of freedom <= 0 for slice.
var = nanvar(a, axis=axis, dtype=dtype, out=out, ddof=ddof,
ads
<xarray.Dataset> Dimensions: (d: 2, sT: 6, time: 3, x: 168, y: 398) Coordinates: * x (x) float64 0.0 0.27 0.54 0.81 ... 44.28 44.55 44.82 45.09 * y (y) float64 0.0 0.27 0.54 0.81 ... 106.4 106.7 106.9 107.2 * time (time) float64 0.005 0.01 0.015 Dimensions without coordinates: d, sT Data variables: displacement (time, y, x, d) float64 -4.206 0.207 ... 0.06631 0.5475 strain (time, y, x, sT) float64 0.001017 -0.006453 ... nan nan std_displacement (time, y, x, d) float64 0.01627 0.0259 ... 0.01252 std_strain (time, y, x, sT) float64 0.002637 0.0338 nan ... nan nan Attributes: unit_time: macro strain step_size: 0.015 unit_position: millimeter window_size: 18 path_dat: /data/FlorianFiguiere/GI06/DIC/DIC/Loading/Speakle_01/nm_... DIC_software: 7D
xarray.Dataset
- d: 2
- sT: 6
- time: 3
- x: 168
- y: 398
- x(x)float640.0 0.27 0.54 ... 44.55 44.82 45.09
array([ 0. , 0.27, 0.54, 0.81, 1.08, 1.35, 1.62, 1.89, 2.16, 2.43, 2.7 , 2.97, 3.24, 3.51, 3.78, 4.05, 4.32, 4.59, 4.86, 5.13, 5.4 , 5.67, 5.94, 6.21, 6.48, 6.75, 7.02, 7.29, 7.56, 7.83, 8.1 , 8.37, 8.64, 8.91, 9.18, 9.45, 9.72, 9.99, 10.26, 10.53, 10.8 , 11.07, 11.34, 11.61, 11.88, 12.15, 12.42, 12.69, 12.96, 13.23, 13.5 , 13.77, 14.04, 14.31, 14.58, 14.85, 15.12, 15.39, 15.66, 15.93, 16.2 , 16.47, 16.74, 17.01, 17.28, 17.55, 17.82, 18.09, 18.36, 18.63, 18.9 , 19.17, 19.44, 19.71, 19.98, 20.25, 20.52, 20.79, 21.06, 21.33, 21.6 , 21.87, 22.14, 22.41, 22.68, 22.95, 23.22, 23.49, 23.76, 24.03, 24.3 , 24.57, 24.84, 25.11, 25.38, 25.65, 25.92, 26.19, 26.46, 26.73, 27. , 27.27, 27.54, 27.81, 28.08, 28.35, 28.62, 28.89, 29.16, 29.43, 29.7 , 29.97, 30.24, 30.51, 30.78, 31.05, 31.32, 31.59, 31.86, 32.13, 32.4 , 32.67, 32.94, 33.21, 33.48, 33.75, 34.02, 34.29, 34.56, 34.83, 35.1 , 35.37, 35.64, 35.91, 36.18, 36.45, 36.72, 36.99, 37.26, 37.53, 37.8 , 38.07, 38.34, 38.61, 38.88, 39.15, 39.42, 39.69, 39.96, 40.23, 40.5 , 40.77, 41.04, 41.31, 41.58, 41.85, 42.12, 42.39, 42.66, 42.93, 43.2 , 43.47, 43.74, 44.01, 44.28, 44.55, 44.82, 45.09])
- y(y)float640.0 0.27 0.54 ... 106.7 106.9 107.2
array([ 0. , 0.27, 0.54, ..., 106.65, 106.92, 107.19])
- time(time)float640.005 0.01 0.015
array([0.005, 0.01 , 0.015])
- displacement(time, y, x, d)float64-4.206 0.207 ... 0.06631 0.5475
array([[[[-4.20594530e+00, 2.06976144e-01], [-4.20948721e+00, 2.08254089e-01], [-4.20932991e+00, 2.06882858e-01], ..., [-4.10964500e+00, 9.08333588e-02], [-4.10803883e+00, 8.99642944e-02], [-4.10943375e+00, 9.02604675e-02]], [[-4.20937866e+00, 2.08470383e-01], [-4.20931744e+00, 2.06875648e-01], [-4.20947531e+00, 2.05892449e-01], ..., [-4.10922209e+00, 9.13687134e-02], [-4.10766432e+00, 8.95101929e-02], [-4.10818096e+00, 8.99212646e-02]], [[-4.20912778e+00, 2.10387726e-01], [-4.20925658e+00, 2.06675262e-01], [-4.20928442e+00, 2.03303567e-01], ..., ... ..., [ 6.77398109e-02, 5.50979176e-01], [ 6.47039030e-02, 5.48907877e-01], [ 6.55764771e-02, 5.50452805e-01]], [[-1.02825167e-04, 5.42845716e-01], [ 2.85964966e-03, 5.45679088e-01], [ 6.60036089e-03, 5.46195953e-01], ..., [ 6.31910133e-02, 5.50182495e-01], [ 6.39983368e-02, 5.48717637e-01], [ 6.53326035e-02, 5.49221592e-01]], [[-2.56862640e-04, 5.41424732e-01], [ 1.76731110e-03, 5.43070736e-01], [ 1.26658630e-02, 5.45309658e-01], ..., [ 6.41742325e-02, 5.49532070e-01], [ 6.38286209e-02, 5.48544130e-01], [ 6.63050079e-02, 5.47457714e-01]]]])
- strain(time, y, x, sT)float640.001017 -0.006453 nan ... nan nan
array([[[[ 1.01675684e-03, -6.45267675e-03, nan, -7.04214013e-03, nan, nan], [-1.26527094e-03, 1.03007846e-02, nan, -1.24408057e-03, nan, nan], [ 5.13501507e-04, 4.21458997e-03, nan, -1.56897413e-03, nan, nan], ..., [ 1.61941654e-03, -1.51111040e-03, nan, -2.42613196e-03, nan, nan], [ 1.62021781e-03, 1.88713041e-03, nan, -1.57717903e-03, nan, nan], [-4.97459937e-03, 1.50041012e-03, nan, -1.38797817e-03, nan, nan]], [[ 8.05190697e-04, -8.67005860e-03, nan, -4.14850292e-03, nan, nan], [-3.98105146e-04, 6.17174420e-03, nan, -1.12370498e-03, nan, nan], [-1.15191924e-04, 8.31007856e-03, nan, -1.62446394e-03, nan, nan], ... [ 2.07765203e-03, 3.10150228e-03, nan, 7.74699277e-04, nan, nan], [-3.24272049e-04, 1.27251937e-03, nan, -5.22654291e-04, nan, nan], [ 4.77457550e-03, 5.11340615e-03, nan, 1.25608630e-03, nan, nan]], [[ 7.31408436e-03, 5.21669629e-03, nan, 3.71247163e-03, nan, nan], [ 2.61312423e-02, 9.56499728e-03, nan, 5.77636925e-03, nan, nan], [ 7.75237908e-03, 2.66104045e-03, nan, 3.59703405e-04, nan, nan], ..., [-2.52067563e-03, 2.78311225e-03, nan, -1.40239132e-03, nan, nan], [ 3.08591007e-03, 1.52526925e-03, nan, -2.91616747e-03, nan, nan], [ 5.86683359e-03, 6.51873202e-03, nan, 8.09027461e-04, nan, nan]]]])
- std_displacement(time, y, x, d)float640.01627 0.0259 ... 0.007625 0.01252
array([[[[1.62714129e-02, 2.59020817e-02], [1.70555005e-02, 2.57651415e-02], [1.72752469e-02, 2.54505901e-02], ..., [7.00059669e-04, 7.10232243e-03], [1.48358471e-04, 6.98237545e-03], [5.01917566e-04, 7.08868836e-03]], [[1.72676731e-02, 2.64051683e-02], [1.72519604e-02, 2.56561191e-02], [1.72785476e-02, 2.52202001e-02], ..., [1.39869733e-04, 7.14636651e-03], [2.90765384e-04, 6.63140297e-03], [2.26356585e-04, 6.73326837e-03]], [[1.69040790e-02, 2.46816248e-02], [1.73088386e-02, 2.50975375e-02], [1.74318180e-02, 2.39379254e-02], ..., ... ..., [6.19997985e-03, 1.01025322e-02], [8.69419825e-03, 1.17402333e-02], [8.12072173e-03, 1.09380639e-02]], [[1.86558977e-04, 1.24662354e-04], [2.72686737e-04, 2.11257295e-04], [2.96682840e-04, 2.53383853e-04], ..., [6.06434152e-03, 1.12119353e-02], [9.08597284e-03, 1.22268187e-02], [7.94308309e-03, 1.17635921e-02]], [[6.51940327e-04, 2.64358861e-04], [2.65687722e-04, 2.01252277e-04], [1.11838006e-02, 9.28017709e-04], ..., [4.90537965e-03, 1.15919872e-02], [9.07348168e-03, 1.22579754e-02], [7.62527679e-03, 1.25202648e-02]]]])
- std_strain(time, y, x, sT)float640.002637 0.0338 nan ... nan nan
array([[[[0.00263657, 0.03379899, nan, 0.01469727, nan, nan], [0.00184324, 0.03018109, nan, 0.00683682, nan, nan], [0.00129522, 0.01255682, nan, 0.00583828, nan, nan], ..., [0.001298 , 0.00114156, nan, 0.00140863, nan, nan], [0.00133013, 0.00167521, nan, 0.00067394, nan, nan], [0.00213649, 0.00158279, nan, 0.00105574, nan, nan]], [[0.00179997, 0.03024851, nan, 0.01287379, nan, nan], [0.0010384 , 0.01972653, nan, 0.00620368, nan, nan], [0.00107244, 0.01852935, nan, 0.00278278, nan, nan], ... [0.00902278, 0.00368003, nan, 0.00157492, nan, nan], [0.00227292, 0.00153392, nan, 0.00082778, nan, nan], [0.00386171, 0.0036393 , nan, 0.00152262, nan, nan]], [[0.00259659, 0.00107874, nan, 0.00176029, nan, nan], [0.02166075, 0.00090625, nan, 0.00125013, nan, nan], [0.00523958, 0.00365301, nan, 0.00121326, nan, nan], ..., [0.0037546 , 0.00300379, nan, 0.00184898, nan, nan], [0.00271864, 0.00102993, nan, 0.00222979, nan, nan], [0.00496499, 0.00383205, nan, 0.00184408, nan, nan]]]])
- unit_time :
- macro strain
- step_size :
- 0.015
- unit_position :
- millimeter
- window_size :
- 18
- path_dat :
- /data/FlorianFiguiere/GI06/DIC/DIC/Loading/Speakle_01/nm_analysis/gdr/
- DIC_software :
- 7D
ads['eqVM_LN']=ads.strain.sT.eqVonMises(lognorm=True)
ads['eqVM']=ads.strain.sT.eqVonMises(lognorm=False)
ads['std_eqVM_LN']=ads.std_strain.sT.eqVonMises(lognorm=True)
ads['std_eqVM']=ads.std_strain.sT.eqVonMises(lognorm=False)
ds['eqVM_LN']=ds.strain.sT.eqVonMises(lognorm=True)
ds['eqVM']=ds.strain.sT.eqVonMises(lognorm=False)
/home/chauvet/Documents/GitGricad/lib-python/xarray_symTensor2d/xarray_symTensor2d/xarray_symTensor2d.py:38: RuntimeWarning: divide by zero encountered in log
deq[j,i,...]=np.log(deq[j,i,...]/med[j,i])
ads
<xarray.Dataset> Dimensions: (d: 2, sT: 6, time: 3, x: 168, y: 398) Coordinates: * time (time) float64 0.005 0.01 0.015 * x (x) float64 0.0 0.27 0.54 0.81 ... 44.28 44.55 44.82 45.09 * y (y) float64 0.0 0.27 0.54 0.81 ... 106.4 106.7 106.9 107.2 Dimensions without coordinates: d, sT Data variables: displacement (time, y, x, d) float64 -4.206 0.207 ... 0.06631 0.5475 strain (time, y, x, sT) float64 0.001017 -0.006453 ... nan nan std_displacement (time, y, x, d) float64 0.01627 0.0259 ... 0.01252 std_strain (time, y, x, sT) float64 0.002637 0.0338 nan ... nan nan eqVM_LN (time, y, x) float64 0.8477 0.7241 ... 0.1806 0.6792 eqVM (time, y, x) float64 0.00794 0.007018 ... 0.005896 std_eqVM_LN (time, y, x) float64 1.437 1.212 0.4679 ... 0.163 0.6228 std_eqVM (time, y, x) float64 0.02651 0.02116 ... 0.002859 0.004528 Attributes: unit_time: macro strain step_size: 0.015 unit_position: millimeter window_size: 18 path_dat: /data/FlorianFiguiere/GI06/DIC/DIC/Loading/Speakle_01/nm_... DIC_software: 7D
xarray.Dataset
- d: 2
- sT: 6
- time: 3
- x: 168
- y: 398
- time(time)float640.005 0.01 0.015
array([0.005, 0.01 , 0.015])
- x(x)float640.0 0.27 0.54 ... 44.55 44.82 45.09
array([ 0. , 0.27, 0.54, 0.81, 1.08, 1.35, 1.62, 1.89, 2.16, 2.43, 2.7 , 2.97, 3.24, 3.51, 3.78, 4.05, 4.32, 4.59, 4.86, 5.13, 5.4 , 5.67, 5.94, 6.21, 6.48, 6.75, 7.02, 7.29, 7.56, 7.83, 8.1 , 8.37, 8.64, 8.91, 9.18, 9.45, 9.72, 9.99, 10.26, 10.53, 10.8 , 11.07, 11.34, 11.61, 11.88, 12.15, 12.42, 12.69, 12.96, 13.23, 13.5 , 13.77, 14.04, 14.31, 14.58, 14.85, 15.12, 15.39, 15.66, 15.93, 16.2 , 16.47, 16.74, 17.01, 17.28, 17.55, 17.82, 18.09, 18.36, 18.63, 18.9 , 19.17, 19.44, 19.71, 19.98, 20.25, 20.52, 20.79, 21.06, 21.33, 21.6 , 21.87, 22.14, 22.41, 22.68, 22.95, 23.22, 23.49, 23.76, 24.03, 24.3 , 24.57, 24.84, 25.11, 25.38, 25.65, 25.92, 26.19, 26.46, 26.73, 27. , 27.27, 27.54, 27.81, 28.08, 28.35, 28.62, 28.89, 29.16, 29.43, 29.7 , 29.97, 30.24, 30.51, 30.78, 31.05, 31.32, 31.59, 31.86, 32.13, 32.4 , 32.67, 32.94, 33.21, 33.48, 33.75, 34.02, 34.29, 34.56, 34.83, 35.1 , 35.37, 35.64, 35.91, 36.18, 36.45, 36.72, 36.99, 37.26, 37.53, 37.8 , 38.07, 38.34, 38.61, 38.88, 39.15, 39.42, 39.69, 39.96, 40.23, 40.5 , 40.77, 41.04, 41.31, 41.58, 41.85, 42.12, 42.39, 42.66, 42.93, 43.2 , 43.47, 43.74, 44.01, 44.28, 44.55, 44.82, 45.09])
- y(y)float640.0 0.27 0.54 ... 106.7 106.9 107.2
array([ 0. , 0.27, 0.54, ..., 106.65, 106.92, 107.19])
- displacement(time, y, x, d)float64-4.206 0.207 ... 0.06631 0.5475
array([[[[-4.20594530e+00, 2.06976144e-01], [-4.20948721e+00, 2.08254089e-01], [-4.20932991e+00, 2.06882858e-01], ..., [-4.10964500e+00, 9.08333588e-02], [-4.10803883e+00, 8.99642944e-02], [-4.10943375e+00, 9.02604675e-02]], [[-4.20937866e+00, 2.08470383e-01], [-4.20931744e+00, 2.06875648e-01], [-4.20947531e+00, 2.05892449e-01], ..., [-4.10922209e+00, 9.13687134e-02], [-4.10766432e+00, 8.95101929e-02], [-4.10818096e+00, 8.99212646e-02]], [[-4.20912778e+00, 2.10387726e-01], [-4.20925658e+00, 2.06675262e-01], [-4.20928442e+00, 2.03303567e-01], ..., ... ..., [ 6.77398109e-02, 5.50979176e-01], [ 6.47039030e-02, 5.48907877e-01], [ 6.55764771e-02, 5.50452805e-01]], [[-1.02825167e-04, 5.42845716e-01], [ 2.85964966e-03, 5.45679088e-01], [ 6.60036089e-03, 5.46195953e-01], ..., [ 6.31910133e-02, 5.50182495e-01], [ 6.39983368e-02, 5.48717637e-01], [ 6.53326035e-02, 5.49221592e-01]], [[-2.56862640e-04, 5.41424732e-01], [ 1.76731110e-03, 5.43070736e-01], [ 1.26658630e-02, 5.45309658e-01], ..., [ 6.41742325e-02, 5.49532070e-01], [ 6.38286209e-02, 5.48544130e-01], [ 6.63050079e-02, 5.47457714e-01]]]])
- strain(time, y, x, sT)float640.001017 -0.006453 nan ... nan nan
array([[[[ 1.01675684e-03, -6.45267675e-03, nan, -7.04214013e-03, nan, nan], [-1.26527094e-03, 1.03007846e-02, nan, -1.24408057e-03, nan, nan], [ 5.13501507e-04, 4.21458997e-03, nan, -1.56897413e-03, nan, nan], ..., [ 1.61941654e-03, -1.51111040e-03, nan, -2.42613196e-03, nan, nan], [ 1.62021781e-03, 1.88713041e-03, nan, -1.57717903e-03, nan, nan], [-4.97459937e-03, 1.50041012e-03, nan, -1.38797817e-03, nan, nan]], [[ 8.05190697e-04, -8.67005860e-03, nan, -4.14850292e-03, nan, nan], [-3.98105146e-04, 6.17174420e-03, nan, -1.12370498e-03, nan, nan], [-1.15191924e-04, 8.31007856e-03, nan, -1.62446394e-03, nan, nan], ... [ 2.07765203e-03, 3.10150228e-03, nan, 7.74699277e-04, nan, nan], [-3.24272049e-04, 1.27251937e-03, nan, -5.22654291e-04, nan, nan], [ 4.77457550e-03, 5.11340615e-03, nan, 1.25608630e-03, nan, nan]], [[ 7.31408436e-03, 5.21669629e-03, nan, 3.71247163e-03, nan, nan], [ 2.61312423e-02, 9.56499728e-03, nan, 5.77636925e-03, nan, nan], [ 7.75237908e-03, 2.66104045e-03, nan, 3.59703405e-04, nan, nan], ..., [-2.52067563e-03, 2.78311225e-03, nan, -1.40239132e-03, nan, nan], [ 3.08591007e-03, 1.52526925e-03, nan, -2.91616747e-03, nan, nan], [ 5.86683359e-03, 6.51873202e-03, nan, 8.09027461e-04, nan, nan]]]])
- std_displacement(time, y, x, d)float640.01627 0.0259 ... 0.007625 0.01252
array([[[[1.62714129e-02, 2.59020817e-02], [1.70555005e-02, 2.57651415e-02], [1.72752469e-02, 2.54505901e-02], ..., [7.00059669e-04, 7.10232243e-03], [1.48358471e-04, 6.98237545e-03], [5.01917566e-04, 7.08868836e-03]], [[1.72676731e-02, 2.64051683e-02], [1.72519604e-02, 2.56561191e-02], [1.72785476e-02, 2.52202001e-02], ..., [1.39869733e-04, 7.14636651e-03], [2.90765384e-04, 6.63140297e-03], [2.26356585e-04, 6.73326837e-03]], [[1.69040790e-02, 2.46816248e-02], [1.73088386e-02, 2.50975375e-02], [1.74318180e-02, 2.39379254e-02], ..., ... ..., [6.19997985e-03, 1.01025322e-02], [8.69419825e-03, 1.17402333e-02], [8.12072173e-03, 1.09380639e-02]], [[1.86558977e-04, 1.24662354e-04], [2.72686737e-04, 2.11257295e-04], [2.96682840e-04, 2.53383853e-04], ..., [6.06434152e-03, 1.12119353e-02], [9.08597284e-03, 1.22268187e-02], [7.94308309e-03, 1.17635921e-02]], [[6.51940327e-04, 2.64358861e-04], [2.65687722e-04, 2.01252277e-04], [1.11838006e-02, 9.28017709e-04], ..., [4.90537965e-03, 1.15919872e-02], [9.07348168e-03, 1.22579754e-02], [7.62527679e-03, 1.25202648e-02]]]])
- std_strain(time, y, x, sT)float640.002637 0.0338 nan ... nan nan
array([[[[0.00263657, 0.03379899, nan, 0.01469727, nan, nan], [0.00184324, 0.03018109, nan, 0.00683682, nan, nan], [0.00129522, 0.01255682, nan, 0.00583828, nan, nan], ..., [0.001298 , 0.00114156, nan, 0.00140863, nan, nan], [0.00133013, 0.00167521, nan, 0.00067394, nan, nan], [0.00213649, 0.00158279, nan, 0.00105574, nan, nan]], [[0.00179997, 0.03024851, nan, 0.01287379, nan, nan], [0.0010384 , 0.01972653, nan, 0.00620368, nan, nan], [0.00107244, 0.01852935, nan, 0.00278278, nan, nan], ... [0.00902278, 0.00368003, nan, 0.00157492, nan, nan], [0.00227292, 0.00153392, nan, 0.00082778, nan, nan], [0.00386171, 0.0036393 , nan, 0.00152262, nan, nan]], [[0.00259659, 0.00107874, nan, 0.00176029, nan, nan], [0.02166075, 0.00090625, nan, 0.00125013, nan, nan], [0.00523958, 0.00365301, nan, 0.00121326, nan, nan], ..., [0.0037546 , 0.00300379, nan, 0.00184898, nan, nan], [0.00271864, 0.00102993, nan, 0.00222979, nan, nan], [0.00496499, 0.00383205, nan, 0.00184408, nan, nan]]]])
- eqVM_LN(time, y, x)float640.8477 0.7241 ... 0.1806 0.6792
array([[[ 0.84766459, 0.7241392 , -0.0630808 , ..., -0.22268452, -0.42350702, 0.08484626], [ 0.72158698, 0.22429081, 0.52464568, ..., -0.53172155, 0.00879408, -0.30543272], [ 0.9466034 , 0.12426811, 0.52041755, ..., 0.53754454, 0.40490797, -0.03759943], ..., [ 1.79728299, -0.19872822, 0.38375751, ..., 0.67265477, 1.17091885, 1.09463713], [ 0.92346172, 0.3970467 , 0.64660031, ..., 0.96917326, 1.13406544, 0.94994246], [ 1.73870949, 1.1070898 , -0.11508621, ..., 1.18969201, 0.65817773, 0.41971774]], [[-0.74388252, -0.32807884, 0.4836103 , ..., 0.27884566, -0.64810438, 0.32788833], [-0.14488105, -0.80461892, -0.14725692, ..., -0.03136114, -0.51510752, -0.39407086], [ 0.25163706, -0.5636288 , -0.1723206 , ..., -0.44478759, 0.28223326, -0.38374415], ... [ 0.13684583, -0.26795091, 0.16091636, ..., 0.1285236 , 0.29320679, 0.90468459], [-0.21401176, -1.00838246, -0.42453701, ..., -0.18407064, -0.29009031, 0.5996306 ], [ 0.09010302, 0.22243236, 0.05699013, ..., 0.90667108, -0.25139936, -0.2632869 ]], [[ 0.18221046, -0.69239157, 0.93678516, ..., -1.71229118, 0.07104759, 1.02918314], [ 0.47246217, -0.8717011 , 0.17274519, ..., -0.62690736, -0.73626406, 0.61566705], [ 1.17537804, 0.37372153, -0.21329524, ..., -0.4513782 , -0.6939926 , -0.49079133], ..., [ 1.36493219, -0.27457637, 0.97850737, ..., 0.07804264, 0.47962571, 0.4202682 ], [ 1.12615062, 1.56120525, 1.10877358, ..., -0.14203137, -1.09051383, 0.4759945 ], [ 0.84175712, 1.86675544, 0.60503553, ..., -0.05447882, 0.18060404, 0.67920128]]])
- eqVM(time, y, x)float640.00794 0.007018 ... 0.005896
array([[[0.00794017, 0.00701752, 0.00319373, ..., 0.0027226 , 0.00222724, 0.0037029 ], [0.00699963, 0.00425699, 0.00574837, ..., 0.0019988 , 0.00343173, 0.00250638], [0.00876594, 0.00385179, 0.00572412, ..., 0.005823 , 0.00509968, 0.00327616], ..., [0.02052315, 0.00278861, 0.00499296, ..., 0.00666537, 0.01097028, 0.01016457], [0.00856541, 0.00505975, 0.00649395, ..., 0.00896604, 0.01057334, 0.00879526], [0.01935557, 0.01029193, 0.00303188, ..., 0.01117817, 0.00656957, 0.00517577]], [[0.00169878, 0.00257465, 0.00579737, ..., 0.00472392, 0.00186954, 0.00496137], [0.00309229, 0.00159868, 0.00308496, ..., 0.00346403, 0.00213547, 0.00241023], [0.00459713, 0.00203433, 0.0030086 , ..., 0.00229104, 0.00473995, 0.00243525], ... [0.00409858, 0.00273421, 0.00419843, ..., 0.00406461, 0.00479226, 0.00883286], [0.00288574, 0.00130397, 0.00233791, ..., 0.00297345, 0.00267434, 0.00651056], [0.00391141, 0.00446481, 0.00378401, ..., 0.00885042, 0.00277984, 0.00274699]], [[0.00358703, 0.00149589, 0.00762855, ..., 0.00053946, 0.00320965, 0.00836701], [0.00479501, 0.00125034, 0.00355324, ..., 0.00159713, 0.00143168, 0.00553328], [0.00968416, 0.00434417, 0.00241529, ..., 0.00190358, 0.0014935 , 0.00183001], ..., [0.01170534, 0.00227172, 0.00795357, ..., 0.00323218, 0.00482948, 0.00455116], [0.00921898, 0.01424375, 0.00906016, ..., 0.00259369, 0.00100461, 0.00481198], [0.00693701, 0.01933407, 0.00547476, ..., 0.00283101, 0.00358127, 0.00589624]]])
- std_eqVM_LN(time, y, x)float641.437 1.212 0.4679 ... 0.163 0.6228
array([[[ 1.43726009, 1.21199794, 0.46786943, ..., -1.27591959, -1.39483861, -1.13080226], [ 1.31956743, 0.82760862, 0.69726227, ..., -2.0768308 , -2.08475514, -1.74759812], [ 1.32497691, 0.67671864, 1.38752582, ..., -1.65212634, -2.05841612, -1.89418651], ..., [ 1.59845078, -0.83444598, -0.82041541, ..., 0.66963535, 1.00120549, 0.8634936 ], [ 1.37241071, 0.2582386 , 0.15381615, ..., 0.05656299, 1.08146041, 1.12471645], [ 1.90318467, 0.89340462, 0.93787656, ..., -0.03960465, 0.88031782, 0.58178789]], [[-0.27167479, -0.38924656, -0.05427524, ..., -0.62748908, -0.42134902, 0.01127588], [-0.47479012, -0.4833306 , -0.39835745, ..., -1.07871325, -1.29686777, -0.54409767], [ 0.01881354, -0.40106817, -0.41975663, ..., -1.0233423 , -1.12196271, -0.89950514], ... [-1.42746508, -1.48905553, -1.55072547, ..., 0.50076041, 0.89974366, 1.67058031], [-0.93229459, -1.21629921, -1.38662939, ..., 0.96889439, 0.37047192, 0.96745948], [-0.30483806, -1.19487503, -1.12546969, ..., 0.84175596, 0.49751284, 0.05378069]], [[-0.52762076, -0.04316387, 1.47551061, ..., -1.03738164, -0.97547756, -0.93257381], [ 1.37377987, 0.06418131, 1.21938035, ..., -1.17907045, -1.69500256, -1.35135284], [ 2.11476829, 1.43015927, 1.45989921, ..., -0.89387381, -1.75879456, -1.17055245], ..., [-0.8876459 , -1.43216715, -0.60190552, ..., 1.35850289, 0.56618253, 0.5074889 ], [-0.42553274, 1.32968053, 1.00220364, ..., 1.00913712, -0.20058104, 0.45210728], [ 0.03018452, 1.78667373, 0.59613321, ..., 0.40686898, 0.1630406 , 0.62279128]]])
- std_eqVM(time, y, x)float640.02651 0.02116 ... 0.004528
array([[[0.02651073, 0.0211637 , 0.01005589, ..., 0.00175834, 0.00156119, 0.00203295], [0.02356722, 0.01440965, 0.01264867, ..., 0.00078935, 0.00078312, 0.00109712], [0.02369505, 0.01239147, 0.02522449, ..., 0.00120703, 0.00080402, 0.00094753], ..., [0.03114769, 0.00273421, 0.00277285, ..., 0.012304 , 0.01714137, 0.01493613], [0.02484608, 0.00815416, 0.00734563, ..., 0.00666488, 0.01857376, 0.01939482], [0.0422445 , 0.01538963, 0.01608949, ..., 0.00605379, 0.01518954, 0.01126925]], [[0.00251813, 0.00223882, 0.00312964, ..., 0.00176421, 0.00216808, 0.00334166], [0.00205526, 0.00203778, 0.00221851, ..., 0.00112354, 0.00090332, 0.00191764], [0.00336695, 0.0022125 , 0.00217154, ..., 0.0011875 , 0.00107598, 0.00134405], ... [0.00079273, 0.00074538, 0.0007008 , ..., 0.00545184, 0.00812493, 0.01756263], [0.00130069, 0.00097912, 0.00082577, ..., 0.00870666, 0.00478586, 0.00869417], [0.00243599, 0.00100032, 0.00107221, ..., 0.00766718, 0.00543417, 0.00348676]], [[0.00143323, 0.00232655, 0.01062343, ..., 0.00086085, 0.00091583, 0.00095598], [0.00959586, 0.00259019, 0.00822297, ..., 0.00074713, 0.00044599, 0.00062889], [0.02013219, 0.01015241, 0.01045887, ..., 0.0009937 , 0.00041843, 0.00075352], ..., [0.00099991, 0.00058006, 0.00133062, ..., 0.00945038, 0.00427906, 0.00403514], [0.00158728, 0.00918188, 0.00661775, ..., 0.00666379, 0.00198768, 0.00381774], [0.00250362, 0.01450111, 0.00440916, ..., 0.00364888, 0.00285934, 0.00452828]]])
- unit_time :
- macro strain
- step_size :
- 0.015
- unit_position :
- millimeter
- window_size :
- 18
- path_dat :
- /data/FlorianFiguiere/GI06/DIC/DIC/Loading/Speakle_01/nm_analysis/gdr/
- DIC_software :
- 7D
Plot the mean strain field¶
plt.figure(figsize=(30,10))
for i in range(3):
plt.subplot(1,3,i+1)
ads.eqVM_LN[i,:,:].plot()
plt.axis('equal')
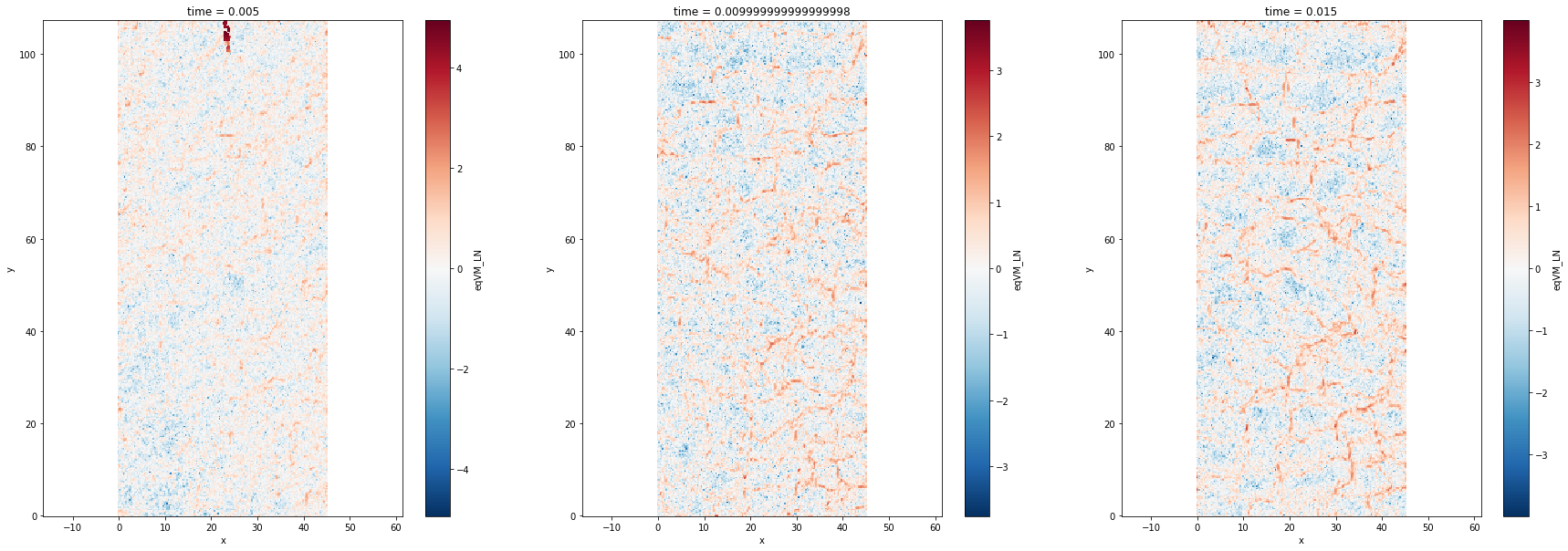
Plot the associated error (STD)¶
plt.figure(figsize=(30,10))
for i in range(3):
plt.subplot(1,3,i+1)
ads.std_eqVM_LN[i,:,:].plot()
plt.axis('equal')
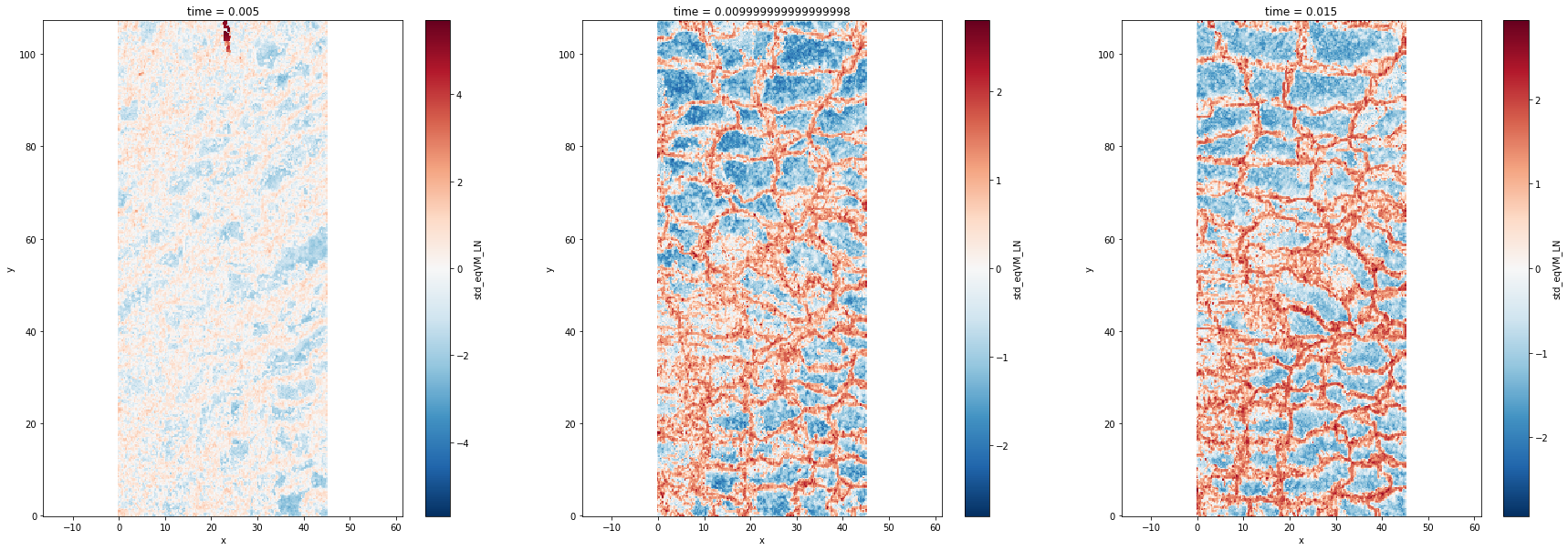
Warning
Here the signal we see on the strain field map is similar to the one on the error map. Therefore we cannot use this for our statistic. The correlation windows used during the DIC is most likely too small